Sean-Bradley Design-Patterns-In-Python: Common GOF Patterns implemented in Python
Table Of Content
- Pattern Program in Python
- GitHub — AmirLavasani/python-design-patterns: Explore design patterns using Python with code…
- A General Purpose Object Factory
- Reverse Alphabet Pyramid Pattern Program
- Factory Design Pattern
- Factory Method Pattern
- Alphabet Diamond Pattern Program
- Hollow Number Diamond Pattern Program
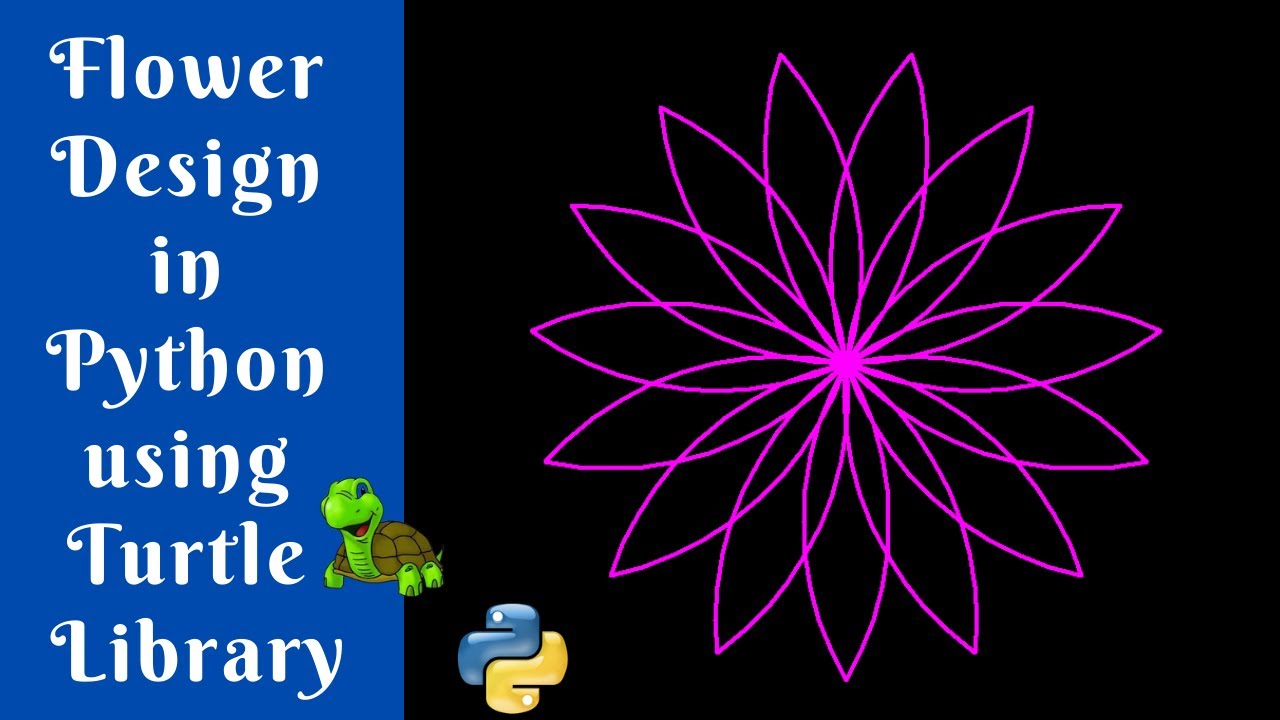
Clients should not be forced to depend upon methods that they do not use. In Rectangle, you’ve provided the .calculate_area() method, which operates with the .width and .height instance attributes. Well, the option here is to add another elif clause to .__init__() and to .calculate_area() so that you can address the requirements of a square shape. You can create circles and rectangles, compute their area, and so on. If you want to read alternate wordings in a quick roundup of these and related principles, then check out Uncle Bob’s The Principles of OOD.
Pattern Program in Python
It establishes a one-to-many relationship between the subject (the object being observed) and the observers (the objects that are interested in the subject’s state). The Observer pattern is useful when you want to decouple the subject from its observers and provide a flexible way to update dependent objects. This class design allows you to create different machines with different sets of functionalities, making your design more flexible and extensible. Here, you pass a pair consisting of a rectangle and a square into a function that calculates their total area. Because the function only cares about the .calculate_area() method, it doesn’t matter that the shapes are different. When someone expects a rectangle object in their code, they might assume that it’ll behave like one by exposing two independent .width and .height attributes.
GitHub — AmirLavasani/python-design-patterns: Explore design patterns using Python with code…
In every case, you’ll have to implement the required interface, which also makes your classes polymorphic. The concept of responsibility in this context may be pretty subjective. Having a single responsibility doesn’t necessarily mean having a single method. Responsibility isn’t directly tied to the number of methods but to the core task that your class is responsible for, depending on your idea of what the class represents in your code. However, that subjectivity shouldn’t stop you from striving to use the SRP.
Recurrent Neural Networks by Example in Python by Will Koehrsen - Towards Data Science
Recurrent Neural Networks by Example in Python by Will Koehrsen.
Posted: Sun, 04 Nov 2018 07:00:00 GMT [source]
A General Purpose Object Factory
The Single Responsibility Principle asks us not to add additional responsibilities to a class so that we don’t have to modify a class unless there is a change to its primary responsibility. This set of principles is not a specific ordered procedure, rather it is a collection of best practices. Duck typing in Python is used to enable support for dynamic typing. The basic concept behind this principle is that you should be concerned about the behavior of an object rather than its type. I build, lead, and mentor software development teams, and for the past few years I've been focusing on cloud services and back-end applications using Python among other languages. You can see that the correct instance is created depending on the specified service type.
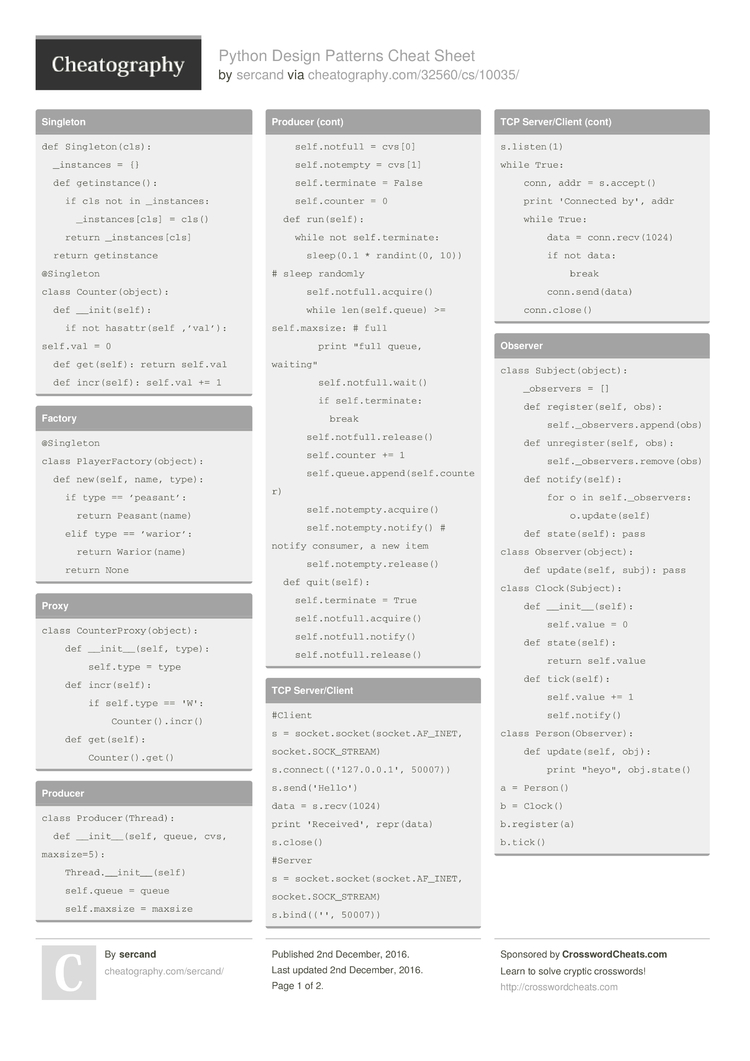
Reverse Alphabet Pyramid Pattern Program
Python's expressive syntax and dynamic nature have led to the birth of certain patterns that might not be as prevalent or even existent in other programming languages. These patterns tackle challenges specific to Python development, offering developers a more Pythonic way to solve problems. The design principle does not just change the direction of the dependency. It suggests decoupling the dependency between the high-level and low-level modules by introducing an abstraction between them.
The registration information is stored in the _creators dictionary. The .get_serializer() method retrieves the registered creator and creates the desired object. If the requested format has not been registered, then ValueError is raised. You can now look at the serialization problem from previous examples and provide a better design by taking into consideration the Factory Method design pattern.
Factory Method Pattern
Decorators are really nice, and we already have them integrated into the language. What I like the most in Python is that using it teaches us to use best practices. It’s not that we don’t have to be conscious about best practices (and design patterns, in particular), but with Python I feel like I’m following best practices, regardless. Personally, I find Python best practices are intuitive and second nature, and this is something appreciated by novice and elite developers alike.
ML Design Pattern #5: Repeatable sampling by Lak Lakshmanan - Towards Data Science
ML Design Pattern #5: Repeatable sampling by Lak Lakshmanan.
Posted: Thu, 07 Nov 2019 08:00:00 GMT [source]
Combine PEP-8 with The Zen of Python (also a PEP - PEP-20), and you’ll have a perfect foundation to create readable and maintainable code. Add Design Patterns and you are ready to create every kind of software system with consistency and evolvability. They're best described as templates for dealing with a certain usual situation.
With this approach, the application code is simplified, making it more reusable and easier to maintain. Maybe you have noticed that none of the design patterns is fully and formally described. You need to “feel” and implement them in the way that best fits your style and needs. Python is a great language and it gives you all the power you need to produce flexible and reusable code. To create a design pattern in Python, you need to understand the problem you’re trying to solve and choose an appropriate design pattern that fits the problem.
Lets you provide a substitute or placeholder for another object. A proxy controls access to the original object, allowing you to perform something either before or after the request gets through to the original object. Lets you attach new behaviors to objects by placing these objects inside special wrapper objects that contain the behaviors. Lets you compose objects into tree structures and then work with these structures as if they were individual objects. Lets you ensure that a class has only one instance, while providing a global access point to this instance. The hollow alphabet diamond pattern is a diamond pattern that is made of alphabets and is hollow inside.
We’ll speak about patterns, and especially Python design patterns, later. Design Patterns is the most essential part of Software Engineering, as they provide the general repeatable solution to a commonly occurring problem in software design. They are only templates that describe how to solve a particular problem with great efficiency. To know more about design patterns basics, refer – Software Design Patterns Tutorial. This tutorial explains the various types of design patterns and their implementation in Python scripting language.
Comments
Post a Comment