Design Patterns in Python: Builder
Table Of Content
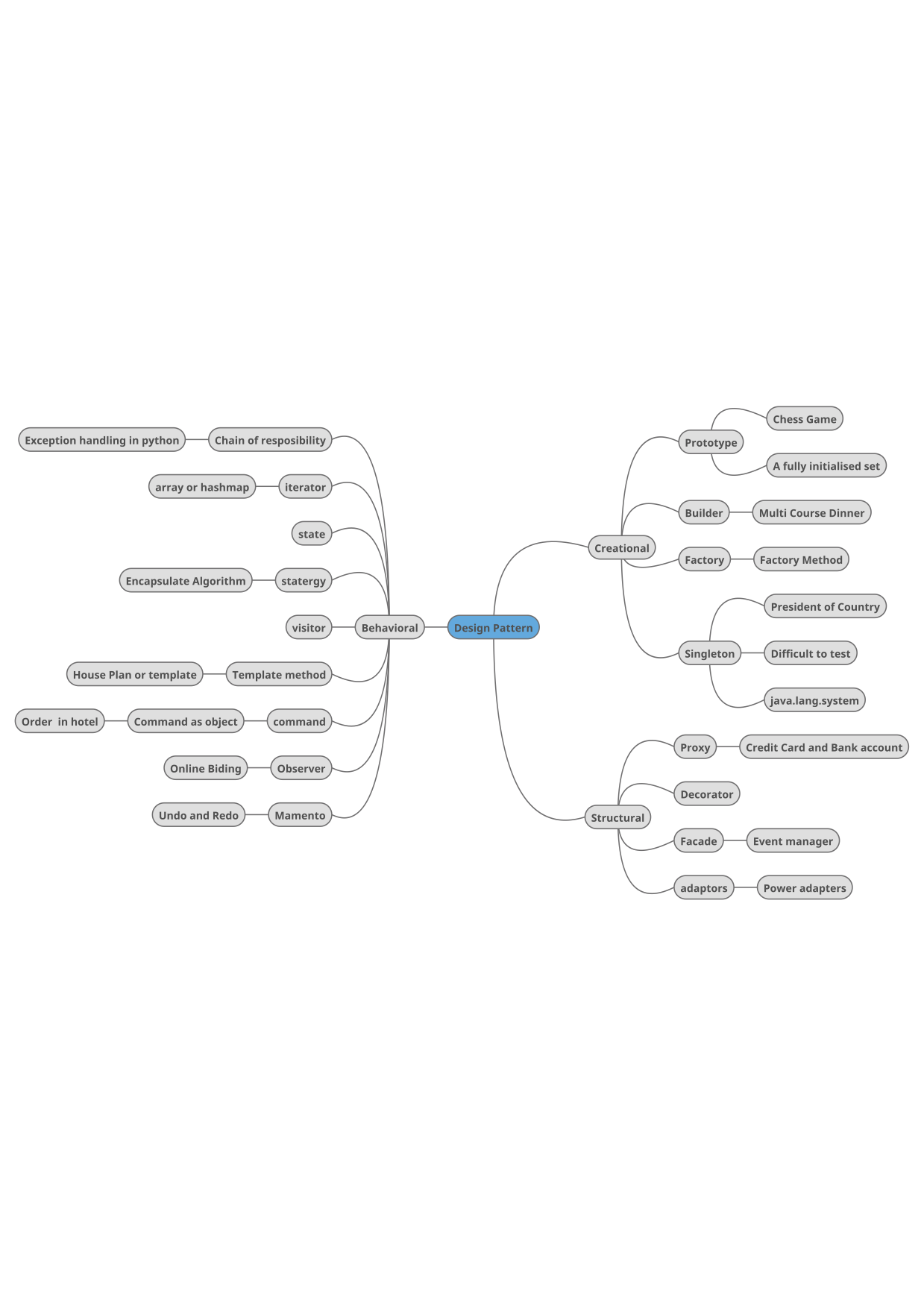
Python’s philosophy is built on top of the idea of well thought out best practices. Python is a dynamic language (did I already said that?) and as such, already implements, or makes it easy to implement, a number of popular design patterns with a few lines of code. Some design patterns are built into Python, so we use them even without knowing. Even a moderately experienced developer could search existing Python code for design patterns and identify them at a glance. Other patterns are not needed due of the nature of the language. Structural Design Patterns deal with assembling objects and classes into larger structures, while keeping those structures flexible and efficient.
Gang of Four: Principles¶
To see Python 2 compatible versions of some patterns please check-out the legacy tag. Add module level description in form of a docstring with links to corresponding references or other useful information. Each of them are an NPC, a common superclass - but they'll have different attributes. The Shopkeeper has charisma, so they can barter better, while the Mage has mana instead of stamina, like the Warrior does.
Data Visualization in Python with Matplotlib and Pandas
Being aware of both the motivations and solutions, you can also avoid accidentally coming up with an anti-pattern while trying to solve a problem. Accompanying real-world examples not only help illustrate the real-life applications of these patterns but also make their implementation in Python more tangible. After reding this article, you should be able to bridge the gap between conceptual understanding and practical application of Python-specific design patterns.
Dynamic Behavior Extension
OOP is one of the most most common programming paradigms, due to its intuitive nature and how well it can reflect the real world. Through OOP, we abstract the physical world into software, allowing us to naturally observe and write code. Each entity becomes an object and these objects can relate to other objects - forming a hierarchy of objects in a system. Also, throughout the book, as each design pattern is discussed and demonstrated using example code, I also introduce new python coding concepts with each new design pattern. So that as you progress through the book and try out the examples, you will also get experience and familiarity with some finer details of programming with python. In this case, clients are classes and subclasses, and interfaces consist of methods and attributes.
When the context manager releases the lock, the other one will enter the if statement and see that the instance has indeed already been created by the other thread. To overcome this, we can make a Builder class that constructs our object and adds appropriate modules to our robot. Instead of a convoluted constructor, we can instantiate an object and add the needed components using functions.
Recognizing Opportunities to Use Factory Method
Why You Should Use Django to Develop Web Apps - Built In
Why You Should Use Django to Develop Web Apps.
Posted: Thu, 10 Dec 2020 08:00:00 GMT [source]
This pattern is useful in scenarios where only one instance of a class is required throughout the application. It helps in managing shared resources and coordinating actions that require a single unified state. However, excessive use of the Singleton pattern can introduce tight coupling and make unit testing more challenging. You’ve learned a lot about the five SOLID principles, including how to identify code that violates them and how to refactor the code in adherence to best design practices. You saw good and bad examples related to each principle and learned that applying the SOLID principles can help you improve your object-oriented design in Python.
How do you create a design pattern in Python?
The Factory Method pattern is commonly used when the exact type of object needed is determined by runtime conditions. Ideally, the design should support adding serialization for new objects by implementing new classes without requiring changes to the existing implementation. Factory Method is a creational design pattern used to create concrete implementations of a common interface. The Singleton design pattern is one of the simplest yet highly beneficial creational design patterns.

Reverse Alphabet Pyramid Pattern Program
If you look closely, you will see that the pattern is a combination of a pyramid pattern and a downward triangle star pattern. Star pattern is a common pattern program created in any programming language. It is a pattern that consists of a series of stars that create some sort of shape. Implementing MVC in Python Implementing MVC in Python is incredibly valuable, especially for web applications. Frameworks like Django and Flask provide MVC architecture out of the box.
In the realm of Python programming, these patterns play a crucial role in enhancing code quality, maintainability, and scalability. The Visitor pattern provides a solution by allowing you to add further operations to objects without having to modify them. It involves creating a visitor class for each operation that needs to be implemented on the elements. The State pattern provides a solution by allowing an object to alter its behavior when its internal state changes.
A direct approach would involve each component knowing about and interacting directly with many other components. This leads to a web of dependencies, making the system hard to maintain and extend. Consider you're building a smart home system where users can control various devices like lights, thermostats, and music players through a central interface. As the system evolves, you'll be adding more devices and functionalities. A naive approach might involve creating a separate method for each action on every device. However, this can quickly become a maintenance nightmare as the number of devices and actions grows.
Let’s start by pointing out that creational patterns are not commonly used in Python. This pattern gives us a way to treat a request using different methods, each one addressing a specific part of the request. You know, one of the best principles for good code is the Single Responsibility principle. For example, today it’s a relational database, but tomorrow it could be whatever, with the interface we need (again those pesky ducks).
Be it creation, structure or behavior, scaling these systems can become very tricky, and with each wrong step - you're deeper entrenched into the problem. This is why Design Patterns are applied and widely-used today. The Observer pattern allows objects to be notified when the state of another object changes.
Comments
Post a Comment